반응형
상품리스트 페이지를 만들어보자
/admin/product/product_list.php
<?php
include $_SERVER["DOCUMENT_ROOT"]."/inc/header.php";
if(!$_SESSION['AUID']){
echo "<script>alert('권한이 없습니다.');history.back();</script>";
exit;
}
$pageNumber = $_GET['pageNumber']??1;//현재 페이지, 없으면 1
if($pageNumber < 1) $pageNumber = 1;
$pageCount = $_GET['pageCount']??10;//페이지당 몇개씩 보여줄지, 없으면 10
$startLimit = ($pageNumber-1)*$pageCount;//쿼리의 limit 시작 부분
$firstPageNumber = $_GET['firstPageNumber'];
$sql = "select * from products where 1=1";
$sql .= $search_where;
$order = " order by pid desc";//마지막에 등록한걸 먼저 보여줌
$limit = " limit $startLimit, $pageCount";
$query = $sql.$order.$limit;
//echo "query=>".$query."<br>";
$result = $mysqli->query($query) or die("query error => ".$mysqli->error);
while($rs = $result->fetch_object()){
$rsc[]=$rs;
}
//전체게시물 수 구하기
$sqlcnt = "select count(*) as cnt from products where 1=1";
$sqlcnt .= $search_where;
$countresult = $mysqli->query($sqlcnt) or die("query error => ".$mysqli->error);
$rscnt = $countresult->fetch_object();
$totalCount = $rscnt->cnt;//전체 갯수를 구한다.
$totalPage = ceil($totalCount/$pageCount);//전체 페이지를 구한다.
if($firstPageNumber < 1) $firstPageNumber = 1;
$lastPageNumber = $firstPageNumber + $pageCount - 1;//페이징 나오는 부분에서 레인지를 정한다.
if($lastPageNumber > $totalPage) $lastPageNumber = $totalPage;
function isStatus($n){
switch($n) {
case -1:$rs="판매중지";
break;
case 0:$rs="대기";
break;
case 1:$rs="판매중";
break;
}
return $rs;
}
?>
<div style="text-align:center;"><h3>제품 리스트</h3></div>
<form method="get" action="<?php echo $_SERVER["PHP_SELF"]?>">
<div class="row g-3" style="padding-bottom:10px;">
<div class="col-md-4">
<select class="form-select" name="cate1" id="cate1" aria-label="Default select example">
<option value="">대분류</option>
<?php
foreach($cate1 as $c){
?>
<option value="<?php echo $c->code;?>"><?php echo $c->name;?></option>
<?php }?>
</select>
</div>
<div class="col-md-4">
<select class="form-select" name="cate2" id="cate2" aria-label="Default select example">
<option value="">중분류</option>
</select>
</div>
<div class="col-md-4">
<select class="form-select" name="cate3" id="cate3" aria-label="Default select example">
<option value="">소분류</option>
</select>
</div>
</div>
<div class="input-group mb-12" style="width:100%;padding-bottom:10px;">
<input class="form-check-input" type="checkbox" name="ismain" id="ismain" value="1">메인
<input class="form-check-input" type="checkbox" name="isnew" id="isnew" value="1">신제품
<input class="form-check-input" type="checkbox" name="isbest" id="isbest" value="1">베스트
<input class="form-check-input" type="checkbox" name="isrecom" id="isrecom" value="1">추천
판매종료일:<input type="text" class="form-control" style="max-width:150px;" name="sale_end_date" id="sale_end_date">
<input type="text" class="form-control" name="search_keyword" id="search_keyword" placeholder="제목과 내용에서 검색합니다." value="<?php echo $search_keyword;?>" aria-label="Recipient's username" aria-describedby="button-addon2">
<button class="btn btn-outline-secondary" type="button" id="search">검색</button>
</div>
</form>
<table class="table table-sm table-bordered">
<thead>
<tr style="text-align:center;">
<th scope="col">사진</th>
<th scope="col">제품명</th>
<th scope="col">가격</th>
<th scope="col">재고</th>
<th scope="col">메인</th>
<th scope="col">신제품</th>
<th scope="col">베스트</th>
<th scope="col">추천</th>
<th scope="col">상태</th>
</tr>
</thead>
<tbody>
<?php
foreach($rsc as $r){
?>
<tr >
<th scope="row" style="width:100px;"><img src="<?php echo $r->thumbnail;?>" style="max-width:100px;"></th>
<td><?php echo $r->name;?></td>
<td style="text-align:right;"><s><?php echo number_format($r->price);?>원</s>
<?php if($r->sale_price>0){?>
<br>
<?php echo number_format($r->sale_price);?>원
<?php }?>
</td>
<td style="text-align:right;"><?php echo number_format($r->cnt-$r->sale_cnt);?>EA</td>
<td style="text-align:center;"><input type="checkbox" name="ismain[<?php echo $r->pid;?>]" id="ismain_<?php echo $r->pid;?>" value="1" <?php if($r->ismain){echo "checked";}?>></td>
<td style="text-align:center;"><input type="checkbox" name="isnew[<?php echo $r->pid;?>]" id="isnew_<?php echo $r->pid;?>" value="1" <?php if($r->isnew){echo "checked";}?>></td>
<td style="text-align:center;"><input type="checkbox" name="isbest[<?php echo $r->pid;?>]" id="isbest_<?php echo $r->pid;?>" value="1" <?php if($r->isbest){echo "checked";}?>></td>
<td style="text-align:center;"><input type="checkbox" name="isrecom[<?php echo $r->pid;?>]" id="isrecom_<?php echo $r->pid;?>" value="1" <?php if($r->isrecom){echo "checked";}?>></td>
<td style="text-align:center;"><?php echo isStatus($r->status);?></td>
</tr>
<?php }?>
</tbody>
</table>
<a href="product_up.php">
<button class="btn btn-primary" type="button">제품등록</button>
</a>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
<script>
$(function(){
$("#sale_end_date").datepicker({ dateFormat: 'yy-mm-dd' });
});
</script>
<?php
include $_SERVER["DOCUMENT_ROOT"]."/inc/footer.php";
?>
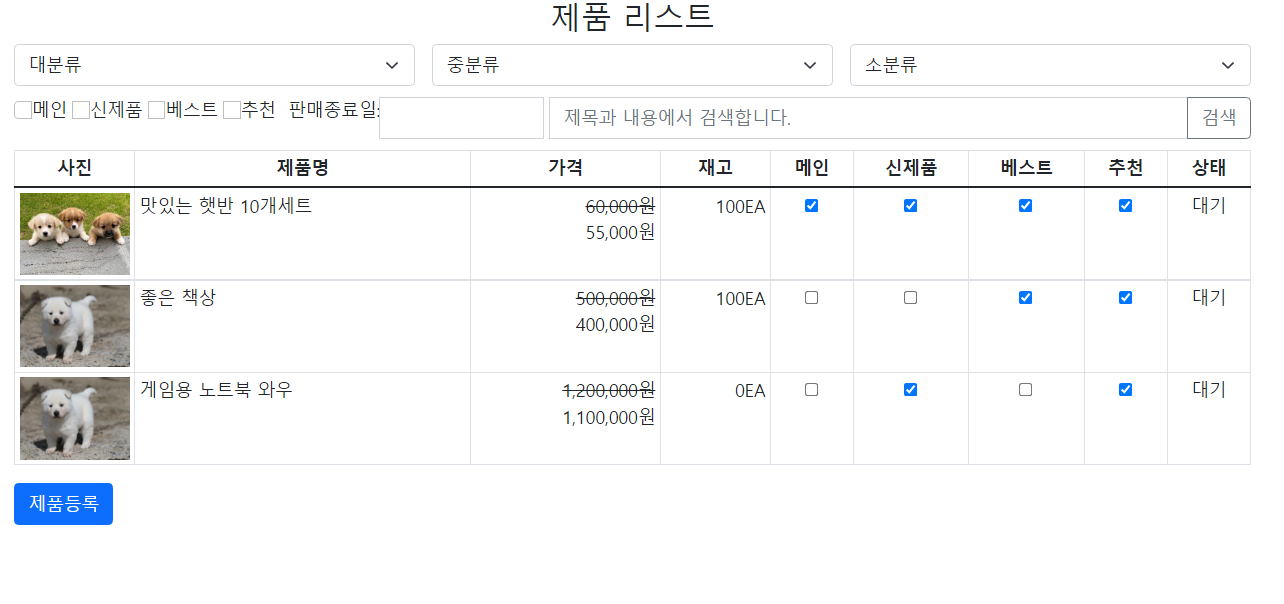
기본적인 것만 해보자. 실제로는 훨씬 더 많은 기능들이 있겠지만 여기서는 검색 몇가지와 간단하게 리스트에서 수정하는걸 해보자. 다음 시간에 검색을 해보자.
반응형
'PHP강좌 > 쇼핑몰만들기강좌' 카테고리의 다른 글
php+mysql 쇼핑몰 만들기 강좌 - #7 상품 리스트에서 한꺼번에 수정하기 (1) | 2022.03.17 |
---|---|
php+mysql 쇼핑몰 만들기 강좌 - #6 상품 검색하기 (2) | 2022.03.16 |
php+mysql 쇼핑몰 만들기 강좌 - #4 상품 등록하기 (0) | 2022.03.03 |
php+mysql 쇼핑몰 만들기 강좌 - #3 관리자 등록하기 (4) | 2022.02.24 |
php+mysql 쇼핑몰 만들기 강좌 - #2 상품 테이블 설계하기 (0) | 2022.02.24 |